Overview
CapTracker is a desktop application. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 10 kLoC.
Summary of Contributions
-
Major Enhancements: added the ability to add, edit, and delete modules.
-
What it does: Allows the user to add, edit, and delete modules in CAPTracker one at a time. name-value paired (-name value) arguments instead of prefix used.
-
Justification: Adding, editing, and deleting is needed to populate the CAPTracker. The name-value pair is also more intuitive for advance CLI users
-
Highlights: name-value pair format was used as advance users are more familiar with it. The way the arguments are tokenize has also been changed.
-
-
Minor enhancement:
-
Make improvements to the APIs:
CommandUtil.java
,ParserUtil.java
, andUniqueModuleList.java
-
-
Code Contributed: Project Code Dashboard - alexkmj
-
Other contributions:
-
Morphing
: Morphed theLogic
andModel
component including its unit test. Introduced theModule
package. -
Organization setup: Created and setup GitHub team organisation.
-
Repository setup: Setup organisation repository. Enable AppVeyor, Codacy, Coveralls, Travis CI, and GitHub Pages and its badges. Create team PR.
-
Manage releases
-
Community
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Adding a module: add
Adds a particular
module entry to the CAPTracker.
Case 1:
Add incomplete
(not graded yet) module.
Format:
add -m CODE -y YEAR -s SEMESTER -c CREDIT
Case 2:
Add completed
(already graded) module.
Format:
add -m CODE -y YEAR -s SEMESTER -c CREDIT -g GRADE
Examples:
Command: add -m MA1521 -y 1 -s 2 -c 4 -g A
Adds a module with:
-
Module code
MA1521
-
Taken in year
1
semester2
-
Worth
4
module credits -
Graded
A
Command: add -m CFG1010 -y 1 -s 1 -c 2 -g CS
Adds a module with:
-
Module code
CFG1010
-
Taken in year
1
semester1
-
Worth
2
module credits -
Graded
CU
Command: add -m CS2103 -y 2 -s 1 -c 4
Adds a module with:
-
Module code
CFG1010
-
Taken in year
2
semester1
-
Worth
4
module credits -
Not completed yet
- Arguments must be in name-value pair format (-name value) - Illegal name or value is not allowed - CODE has to be specified- YEAR has to be specified- SEMESTER has to be specified- CREDIT has to be specified- GRADE has to be specified if it is completed- Module should not exist in CAPTracker |
Editing a module : edit
Edits fields of a particular
module entry in the CAPTracker.
Case 1:
Only one
module entry have the specified target module code.
Pretty Print Format:
edit -t TARGET_CODE [-m NEW_CODE ] [-y NEW_YEAR ] [-s NEW_SEMESTER] [-c NEW_CREDIT ] [-g NEW_GRADE ]
Case 2:
Two or more
module entries has the specified target module code. (E.g. Retook
the module)
Pretty Print Format:
edit -t TARGET_CODE -e TARGET_YEAR -z TARGET_SEMESTER [-m NEW_CODE ] [-y NEW_YEAR ] [-s NEW_SEMESTER] [-c NEW_CREDIT ] [-g NEW_GRADE ]
Examples:
Command: edit -t MA1521 -g A+
Change grade of MA1521
to A+
.
Command: edit -t CFG1010 -m ST2334 -c 4
Change module credit to 4
and module code to ST2334
.
Deleting a module : delete
Deletes a particular
module entry in the CAPTracker.
Case 1:
Only one
module entry have the specified target module code.
Format:
delete -t TARGET_CODE
Case 2:
Two or more
module entries has the specified target module code. (E.g. Retook
the module)
Format:
delete -t TARGET_CODE -e TARGET_YEAR -z TARGET_SEMESTER
Examples:
Command: delete -t CS2103
Deletes the only CS2103
module.
Command: delete -t CS2103 -e 3 -z 2
Deletes CS2103
taken in year 3
semester 2
.
In this specific case, CS2103
was retaken and there exist multiple entries
of it.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Logic component
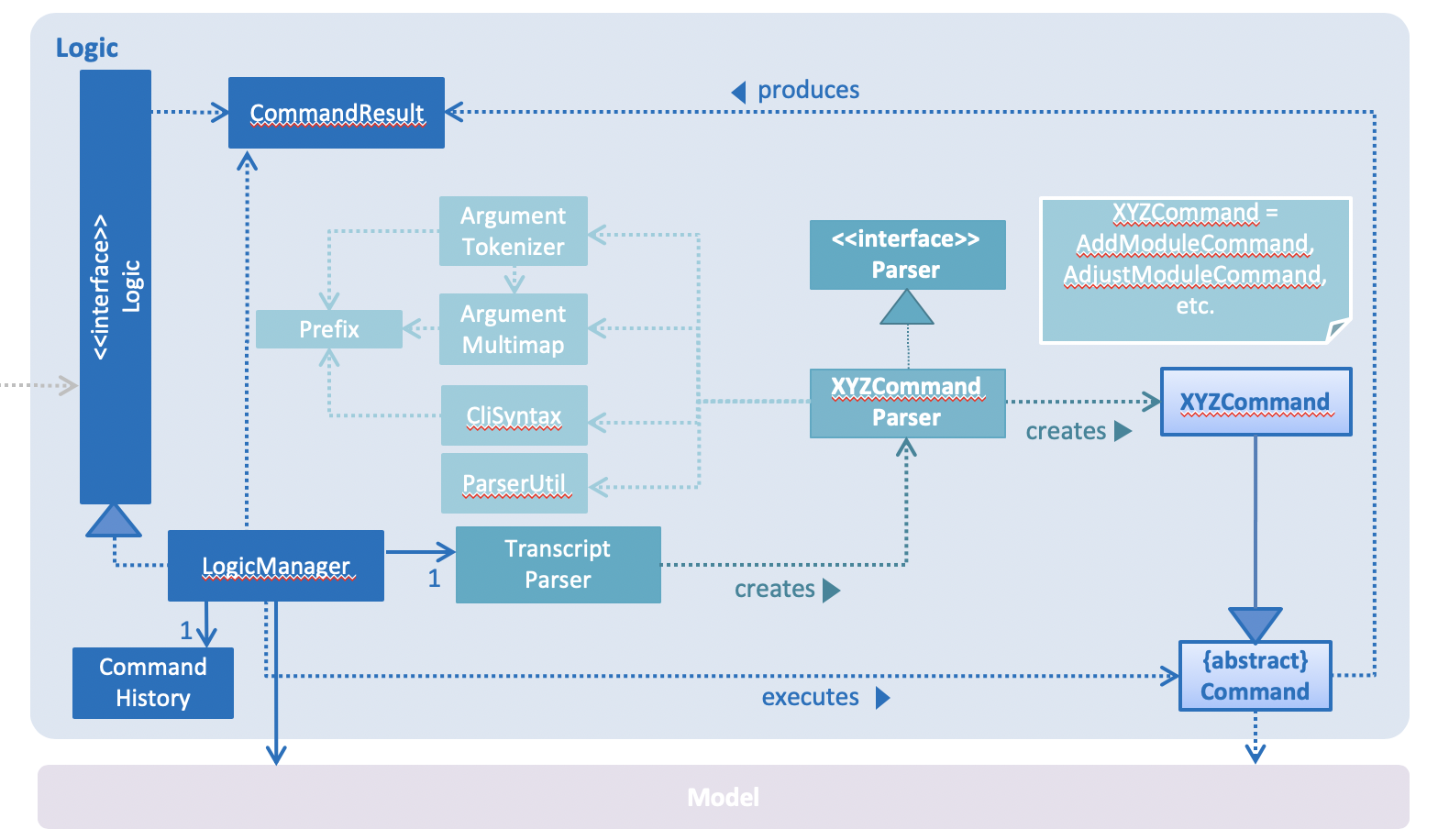
API :
Logic.java
-
Logic
uses theTranscriptParser
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding a module) and/or raise events. -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
.
Given below is the Sequence Diagram for interactions within the Logic
component for the
execute("delete -t CS1231")
API call.
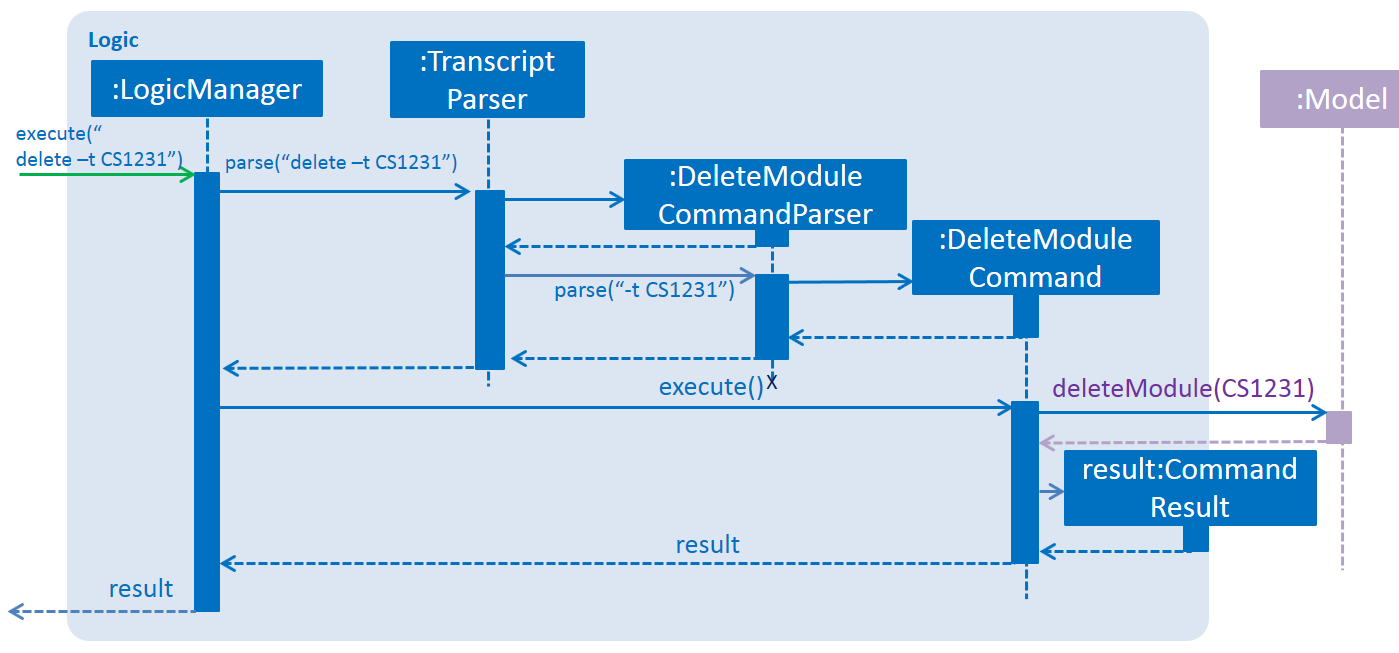
delete -t CS1231
Command