Overview
CapTracker is a desktop application. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 10 kLoC.
Summary of contributions
-
Major enhancement: Implemented the CAP & Target Grade Calculation feature in Model.
-
What it does: Calculates the current CAP of the user and also calculate the target grades the user have to achieve in order to achieve their CAP Goal.
-
Justification: This is one of the must-have User Story of the application, to allow users to verify if they are able to graduate with their desired CAP Goal
-
Highlights:
-
Calculation of target grades are triggered when changes are made to the list of modules in the transcript and updated on the UI.
-
Users may further adjust the target grade and obtain a updated list of target grades
-
-
-
Minor enhancement:
-
Added
adjust
andgoal
command in Logic
-
-
Code Contributed: https://nus-cs2103-ay1819s1.github.io/cs2103-dashboard/#=undefined&search=jeremiah-ang
-
Other contributions:
-
Project management:
-
Maintained issue tracker, milestones and review/merger of pull requests.
-
-
Documentation:
-
Updated existing content of the Model Section of Developer Guide
-
Updated Use Cases to follow the proper format
-
Added Sequence Diagrams for Target Grade and CAP Calculations
-
Added Activity Diagram for Target Grade Calculations
-
Updated User Guide for
goal
andadjust
commands -
Added Manual Testing Guide for Target Grade and CAP Calculations
-
-
Community:
-
PRs reviewed (with non-trivial review comments): #76, #78, #102, #133, #135, #190, #216
-
-
Contributions to the User Guide
Setting Cap Goal : goal
Set the CAP goal you want to achieve.
Format: goal CAP_GOAL
Examples:
-
goal 4.5
Update your CAP goal to 4.5
Adjusting target goals: adjust
Removal of adjustment will be made available in v1.5. |
Adjust the grade of an incomplete module
Format:
-
Module code is unique:
adjust MODULE_CODE GRADE
-
Otherwise:
adjust MODULE_CODE YEAR SEM GRADE
Examples:
-
adjust CS2103 A
Adjusts the grade with module code CS2103 to have grade A -
adjust CS2103 1 1 A
Adjusts the grade with module code CS2103 taken in year 1 sem 1 to have grade A
Contributions to the Developer Guide
Model component
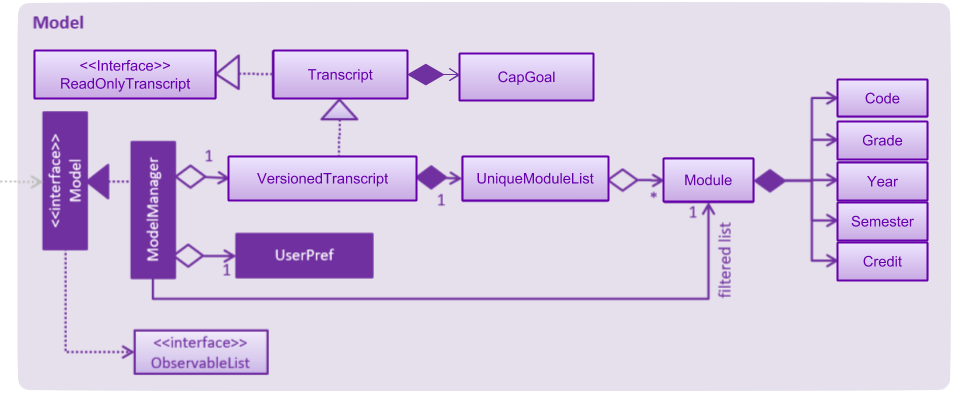
API : Model.java
The Model
,
-
stores a
UserPref
object that represents the user’s preferences. -
stores the Transcript data.
-
exposes an unmodifiable
ObservableList<Module>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. -
does not depend on any of the other three components.
-
provides filter function to filter
Module
with different kind ofGrade
CAP & Target Grades Calculation
The two calculations are triggered upon an change to the list of modules in Transcript
i.e. add/update/delete.
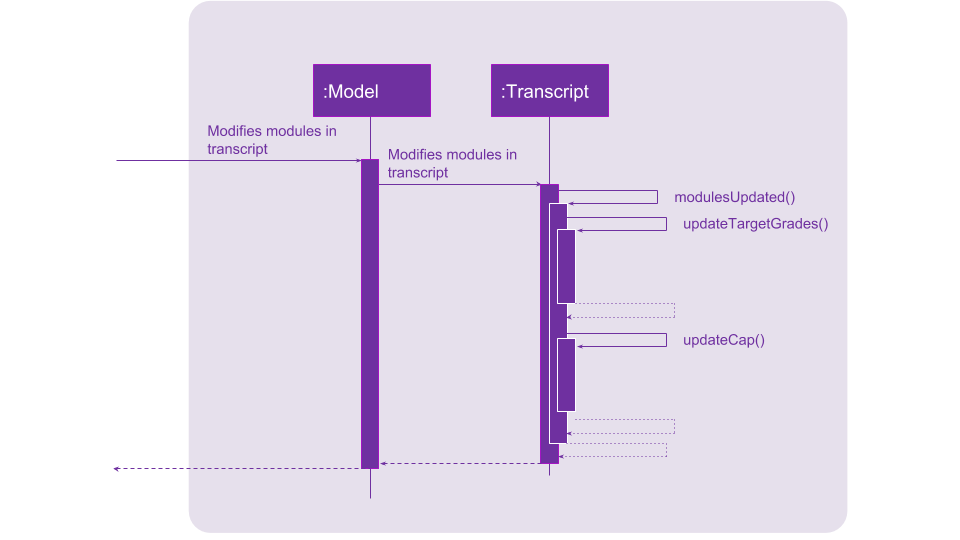
CAP Calculation
The CAP calculation is handled by Transcript
.
The pseudo-code for CAP is the following:
all_points <- sum(credits(m) * points(m) for all completed modules m)
all_credits <- sum(credits(m) for all completed modules m)
CAP <- all_points/all_credits
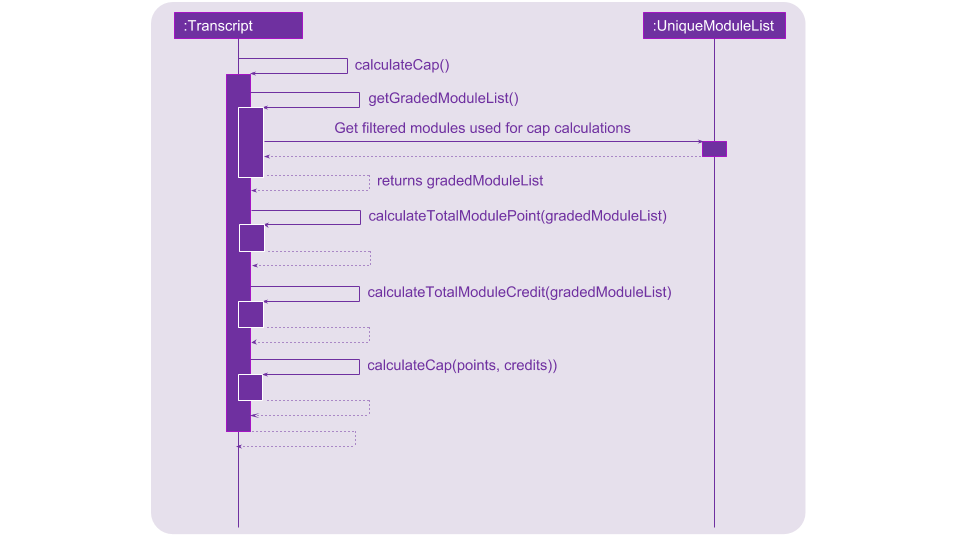
CAP Calculation is triggered by:
Target Grades Calculation
The target Grade
calculation is facilitated by Transcript
.
The returned list of modules with target Grade
assures the following properties:
-
Reducing the
Grade
of any proposed target will result in the increase of another. -
If
x
is the minimumGrade
required when assigned to all modules to obtain the desired CAP Goal, none of the proposed targetGrade
will be greater thanx
i.e. if assigning
B+
to all module is the minimal requirement to obtain the desired CAP Goal, none of the proposed targetGrade
will beA-
or above.
Below is the pseudo-code for Target Grade Calculation:
CG <- CAP goal of user.
TC <- total credit of completed and incomplete modules.
PO <- total points achieved from completed and adjusted modules.
P <- CG * TC - PO // total points needed to achieve from incomplete modules.
mc_remaining <- sum of module credit of all incomplete modules
accumulated_points <- 0
for every incomplete Module m:
avg_point_per_mc <- (P - accumulated_points) / mc_remaining
target(m) <- ceiling(avg_point_per_mc)
mc_remaining <- mc_remaining - credits(m)
accumulated_points <- accumulated_points + (credits(m) * target(m))
This sequence diagram shows the interaction of the different classes involved in the process of creating a new Module with updated target grade
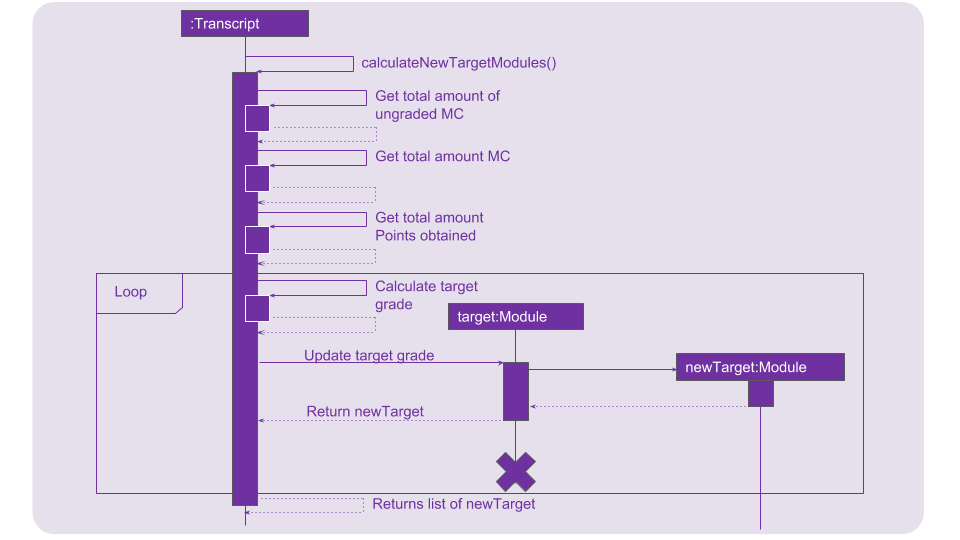
And below the activity diagram to further illustrate several exceptional cases.
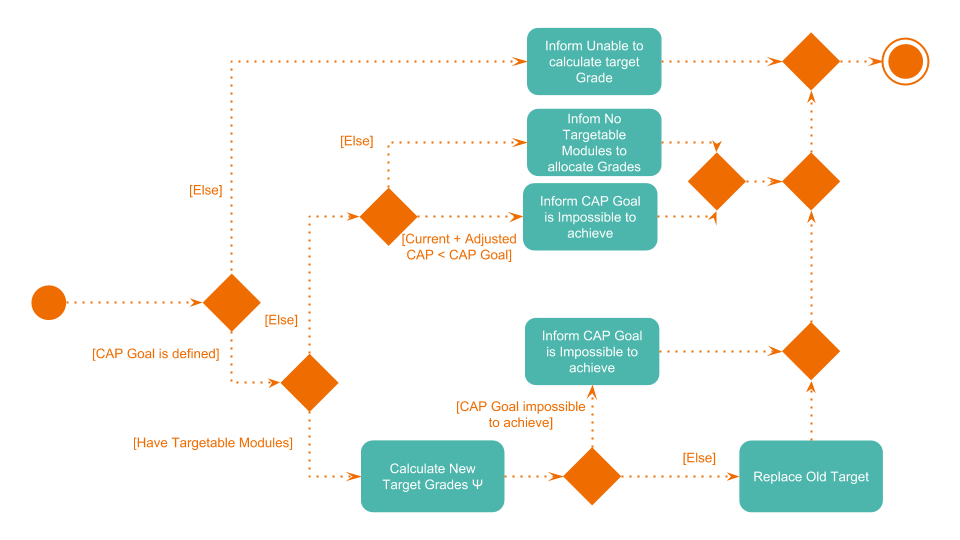
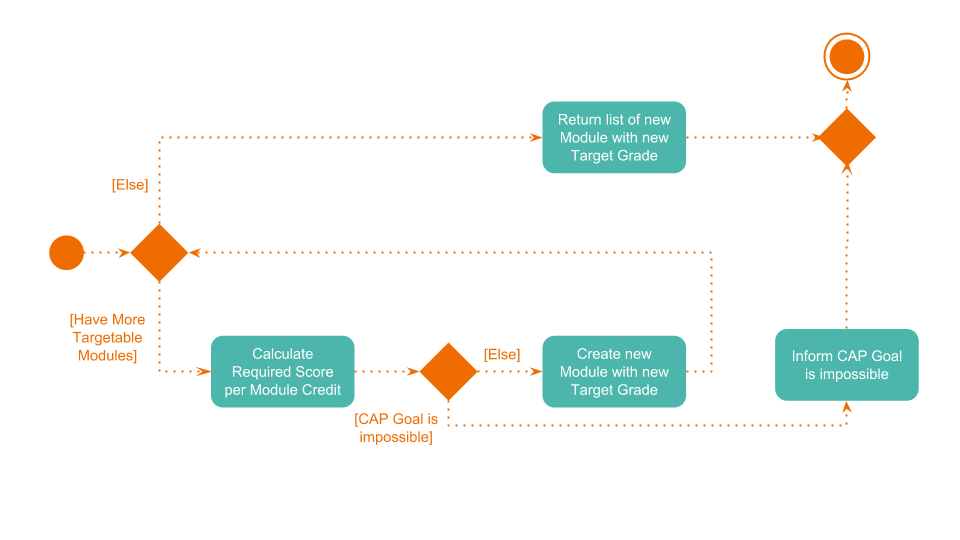
Use case: [UC1] Calculating current CAP
MSS
-
User enter modules
-
System recalculates CAP
-
System displays CAP
Use case ends.
Extensions
-
1a. User enters invalid parameters
-
1a1. System shows an
Invalid entry
error messageUse case ends.
-
-
1b. User enters duplicate Module
-
1b1. System shows an
Duplicate Module
error messageUse case ends
-
Use case: [UC2] View grades needed to achieve CAP goal
MSS
-
User enters completed Modules
-
User enters incomplete Modules
Step 1-2 are repeated until user is satisfied.
-
User enter CAP goal
-
System calculated target grades
-
System displays target grades for ungraded modules
Use case ends.
Extensions
-
3a. CAP goal is invalid
-
3a1. System shows an
Invalid CAP Goal
error messageUse case ends.
-
-
4a. There are no incomplete Modules and current CAP is lesser than CAP Goal
-
4a1. Go to step
5a
Use case ends.
-
-
5a. CAP goal is not achievable
-
5a1. System inform that it is not achievable
Use case ends.
-
Use case: [UC3] Updating target grades
Pre-condition: [UC2]
completed
MSS
-
User modify modules entries
-
System recalculates target grades for ungraded modules
-
System displays new target grades for ungraded modules
Use case ends.
Extensions
-
2a. CAP goal is not achievable with new set of modules
-
2a1. System inform that it is not achievable
Use case ends.
-
Use case: [UC5] Loading saved modules
Pre-conditions: [UC4]
completed
MSS
-
User restarts the application
-
User list entered modules
-
System displays saved modules
Use case ends
Use case: [UC6] Adjusting target grades
Pre-conditions:
-
[UC2]
completed -
There are targets given to incomplete modules
MSS
-
User adjust target
-
System recalculates target grades for remaining ungraded modules
-
System displays new target grades for remaining ungraded modules
Extensions
-
2a. CAP goal is not achievable with new set of modules
-
2a1. System inform that it is not achievable
Use case ends.
-